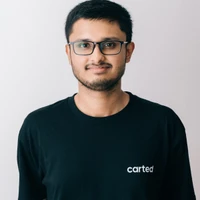
sayakpaul/gemma-2b-sft-qlora-no-robots
Updated
•
44
prompt
stringlengths 43
1.01k
| prompt_id
stringlengths 64
64
| messages
listlengths 2
2
| category
stringclasses 1
value |
---|---|---|---|
This Java function below is not working as I expected, it's supposed to go through an array of numbers and if it finds a number in the array that is divisible by 2 with a remainder of 0, then it adds it to a new array and returns the new array at the end. Can you help debug the function and explain how why it's not working as expected?
public static List checkForEvens(int [] nums) {
ArrayList anwer = new ArrayList<Integer>();
for(int i = 0; i < nums.length; i++) {
if(nums[i] / 2 == 0) {
answer.add(nums[i]
}
}
} | 645c6de54016df90ea64d9b72a1686de2c682467cc0988ed2b8379e4af3a3b69 | [
{
"content": "This Java function below is not working as I expected, it's supposed to go through an array of numbers and if it finds a number in the array that is divisible by 2 with a remainder of 0, then it adds it to a new array and returns the new array at the end. Can you help debug the function and explain how why it's not working as expected?\n\npublic static List checkForEvens(int [] nums) {\n ArrayList anwer = new ArrayList<Integer>();\n for(int i = 0; i < nums.length; i++) {\n if(nums[i] / 2 == 0) {\n answer.add(nums[i]\n } \n }\n}",
"role": "user"
},
{
"content": "Certainly! The function 'checkForEvens' iterates through an array and if a number in the array is divisible by 2, then it gets added to 'answer' which is later returned.\n\nThe reason the function may not be returning the expected result is because of the if statement, 'if (nums[i] /2 == 0)'. The statement is looking for numbers that get divided by 2 in the array and equal 0, which doesn't align with what you expect the function to do. \n\nInstead, you want to use the modulo operator to check for the remainder of a number that is being divided by 2. If a number is divided by 2 and returns a remainder of 0, then we can assume that it's an even number. So, the small change of 'if (nums[i] % 2 == 0)' should solve your issue. \n\nI've rewritten the function below with the correction.\n\npublic static List checkForEvens(int [] nums) {\n ArrayList anwer = new ArrayList<Integer>();\n for(int i = 0; i < nums.length; i++) {\n if(nums[i] % 2 == 0) {\n answer.add(nums[i]\n } \n }\n}",
"role": "assistant"
}
] | Coding |
How do I listen for an event in Discord.js? | 757b00e4f2279890ebb97605a867e4309c29fce4ddfd2035ff568c7be0bb6b6d | [
{
"content": "How do I listen for an event in Discord.js?",
"role": "user"
},
{
"content": "You can listen for an event in Discord.js by using the Client#on method:\n\nconst { Events, Client } = require(\"discord.js\");\nconst client = new Client();\nclient.on(Events.ClientReady, c => {\n console.log(\"Client is ready.\");\n});",
"role": "assistant"
}
] | Coding |
I want to make a video game in Python! Can you show me how to make a game window with a player character in python? | 2526b13cafe55ba808ebe720cc2ebfce41f9e0a12b7512ffe6dbf7eb41a559c5 | [
{
"content": "I want to make a video game in Python! Can you show me how to make a game window with a player character in python?",
"role": "user"
},
{
"content": "Sure! We'll use the Pygame software package to simplify the game structure, which you may need to install separately.\n\nimport pygame\npygame.init()\n\n# Set the width and height of the screen\nsize = (800, 600)\nscreen = pygame.display.set_mode(size)\n\n# Set the title of the window\npygame.display.set_caption(\"A Basic Game in Python!\")\n\n# Load the background image\nbackground_image = pygame.image.load(\"background.png\").convert()\n\n# Load the character image\ncharacter_image = pygame.image.load(\"character.png\").convert_alpha()\n\n# Set the beginning position of the character\ncharacter_position = [100, 100]\n\n# Main game loop starts here\nrunning=True\nwhile running:\n # Handle quit event\n for event in pygame.event.get():\n if event.type == pygame.QUIT:\n running=False\n\n # Draw the background image\n screen.blit(background_image, [0, 0])\n\n # Draw the character sprite at the beginning position\n screen.blit(character_image, character_position)\n\n # Update the screen\n pygame.display.flip()\n\n# Quit the game\npygame.quit()\n\nThis script creates a window with a resolution of 800x600, draws a background and a character, and then runs until the user quits. This is a good base for your new Python video game, to which you can add various features depending on your goals for the game. \n\nNote that both the background and character image should be in the same directory as the game file.\n\nHappy PyGaming!",
"role": "assistant"
}
] | Coding |
May you explain what's going on in this snippet of code? I am not familiar with it.
.action-panel{
@apply fixed left-0 flex flex-col top-72 space-y-2;
}
.action-button{
@apply bg-white relative top-2 py-2 text-center px-6;
} | 525e28a56ceec00670ad602d6553ffdf08501e8a0ca7ea10027a2136bf135d54 | [
{
"content": "May you explain what's going on in this snippet of code? I am not familiar with it.\n\n.action-panel{\n @apply fixed left-0 flex flex-col top-72 space-y-2;\n}\n.action-button{\n @apply bg-white relative top-2 py-2 text-center px-6;\n}",
"role": "user"
},
{
"content": "The provided snippet is using Tailwind CSS to define styles for two classes. The action-panel class is a fixed-position flex column with no left-hand spacing and some vertical spacing between column elements. The action-button class has a background color of white, relative position, center-aligned text, and some vertical and horizontal padding.",
"role": "assistant"
}
] | Coding |
I have an list of numbers in a variable named x. Can you show me how to count the elements in the array, by printing out each element followed by a colon followed by a count? Like 4: 10 if 4 appears 10 times. I'm a Python programmer.
All I want is the code. You can use an example with x = [3, 5, 3, 3, 5, 1]
| 4bdf2305eb042d59d61126bd3018e2a216c7e46b98ba4d8be57f5ad86b82734c | [
{
"content": "I have an list of numbers in a variable named x. Can you show me how to count the elements in the array, by printing out each element followed by a colon followed by a count? Like 4: 10 if 4 appears 10 times. I'm a Python programmer.\n\nAll I want is the code. You can use an example with x = [3, 5, 3, 3, 5, 1]\n",
"role": "user"
},
{
"content": "from collections import Counter\n \nx = [3, 5, 3, 3, 5, 1]\n \ncounts = Counter(x)\n \nfor n in counts.keys():\n print(\"%d: %d\" % (n, counts[n]))",
"role": "assistant"
}
] | Coding |
May you write me a simple application in Vue 3 where there is a number and two buttons that increment and decrement that number? | 9f2696fa31f6f52d177f0acde38b92505c990b9a2cb440af41bababe680ca217 | [
{
"content": "May you write me a simple application in Vue 3 where there is a number and two buttons that increment and decrement that number?",
"role": "user"
},
{
"content": "Of course, below is an example of a bare-bones Vue application that has a number and two buttons that increment and decrement that number using inline event handling....\n\n<html lang=\"en\">\n<head>\n <meta charset=\"UTF-8\">\n <meta name=\"viewport\" content=\"width=device-width, initial-scale=1.0\">\n <title>Learning Vue</title>\n <script src=\"https://unpkg.com/vue@3.0.0\"></script>\n</head>\n<body>\n\n <div id=\"app\">\n <p>{{ num }} years old</p>\n \n <button v-on:click=\"num++\">Increase age</button>\n <button @click=\"num--\">Decrease age</button>\n </div>\n\n\n <script src=\"app.js\"></script>\n</body>\n</html>\n\nWith the accompanying Javascript file looking like this ...\n\nconst app = Vue.createApp({\n data() {\n return {\n num: 45\n }\n }\n})\n\napp.mount('#app')",
"role": "assistant"
}
] | Coding |
I need a Python script that takes the list below and calculates how many players are available for each day. Then find the day with the highest amount of player availability and show me the names of each player that is available for that day.
players = [{'name': 'Bob', 'availability': ['Monday', 'Tuesday', 'Friday']}, {'name': 'Ben Tyson', 'availability': ['Tuesday', 'Thursday', 'Saturday']}, {'name': 'Joyce Sellers', 'availability': ['Monday', 'Wednesday', 'Friday', 'Saturday']}, {'name': 'Milly Rock', 'availability': ['Wednesday', 'Thursday', 'Sunday']}, {'name': 'Stephen Adams', 'availability': ['Thursday', 'Saturday']}, {'name': 'Joe Lynn', 'availability': ['Monday', 'Thursday']}, {'name': 'Latasha Bryan', 'availability': ['Monday', 'Sunday']}, {'name': 'Crystal Brewer', 'availability': ['Thursday', 'Friday', 'Saturday']}, {'name': 'JB Jr.', 'availability': ['Tuesday', 'Wednesday', 'Thursday', 'Sunday']}, {'name': 'Michel Trujillo', 'availability': ['Monday', 'Tuesday', 'Wednesday']}] | a2cc15697d3bad72aa51063c3d8d42753ee59d965111cf28aecc97dded3d8376 | [
{
"content": "I need a Python script that takes the list below and calculates how many players are available for each day. Then find the day with the highest amount of player availability and show me the names of each player that is available for that day. \n\nplayers = [{'name': 'Bob', 'availability': ['Monday', 'Tuesday', 'Friday']}, {'name': 'Ben Tyson', 'availability': ['Tuesday', 'Thursday', 'Saturday']}, {'name': 'Joyce Sellers', 'availability': ['Monday', 'Wednesday', 'Friday', 'Saturday']}, {'name': 'Milly Rock', 'availability': ['Wednesday', 'Thursday', 'Sunday']}, {'name': 'Stephen Adams', 'availability': ['Thursday', 'Saturday']}, {'name': 'Joe Lynn', 'availability': ['Monday', 'Thursday']}, {'name': 'Latasha Bryan', 'availability': ['Monday', 'Sunday']}, {'name': 'Crystal Brewer', 'availability': ['Thursday', 'Friday', 'Saturday']}, {'name': 'JB Jr.', 'availability': ['Tuesday', 'Wednesday', 'Thursday', 'Sunday']}, {'name': 'Michel Trujillo', 'availability': ['Monday', 'Tuesday', 'Wednesday']}]",
"role": "user"
},
{
"content": "I can assist you with that. Here is a Python script that calculates the number of players available for each day, calculates the day with the most available players, and prints out the player names who are available that day.\n\n```Python\n# Calculate how many players are available for each day\ndef populate_availability(players_list, availability):\n for player in players_list:\n for day in player['availability']:\n availability[day] += 1\n\n# Calculate the day with the most available players\ndef find_best_day(availability_table_table):\n highest_num = 0\n best_day = ''\n for key, value in availability_table.items():\n if value > highest_num:\n highest_num = value\n best_day = key\n return best_day\n\n# Calculate the player names that are available on the chosen day\ndef available_on_day(players_list, day):\n players_available = []\n for player in players_list:\n if day in player['availability']:\n players_available.append(player['name'])\n return players_available\n \ndays_available = {'Monday': 0, 'Tuesday': 0, 'Wednesday': 0, 'Thursday': 0, 'Friday': 0, 'Saturday': 0, 'Sunday': 0}\npopulate_availability(players, days_available)\ngame_day = find_best_day(days_available)\nattending_game_day = available_on_day(players, game_day)\n\nprint(attending_game_day)\n# Output: ['Ben Tyson', 'Milly Rock', 'Stephen Adams', 'Joe Lynn', 'Crystal Brewer', 'JB Jr.']\n```\n\nThis script has 3 functions. \n\n1. populate_availability(players_list, availability) - This calculates how many players are available for each day. The first parameter `players_list` accepts a list of players. The second parameter `availability` accepts a dictionary of the days of the week paired with the availability count.\n2. find_best_day(availability_table) - This calculates the day with the most available players. The parameter `availability_table` accepts a dictionary of the days of the week paired with the availability count.\n3. available_on_day(players_list, day) This calculates the player names that are available on the chosen day\n\nAfter the functions are defined, a default dictionary named `days_available` is created. Then each function is called and the final result containing the names of each player that is available for the game day is displayed.",
"role": "assistant"
}
] | Coding |
Could you please write a program that will give me a place to enter a string then show me the unicode of it? I use Python. | bc3916b72a42f1e5762f0b6ec42187c26c4be4e1bdfc91ee538d2ca24a88e4d1 | [
{
"content": "Could you please write a program that will give me a place to enter a string then show me the unicode of it? I use Python.",
"role": "user"
},
{
"content": "This program will accept a string input and output the unicode characters by converting it with `ord()`:\n\n# Get an input string\nmy_string = input(\"Enter the string to convert to Unicode: \")\n\n# Iterate over each character in the string, convert, and print\nfor character in my_string:\n print(ord(character))\n\nAt the beginning of this program, an `input()` statement will prompt you to enter the text you want converted to Unicode. Because the `ord()` function will only accept one character at a time, we can't simply pass the input string into `ord()`. Instead, we use a `for` loop to iterate over each character in the string and print it with a `print()` statement around the `ord()` conversion. If we did not use a `for` loop and tried to enter the entire string into, `ord()` we would receive a TypeError.",
"role": "assistant"
}
] | Coding |
I want my Python code not to error out if it runs into an error and to follow a different line of code. How do I make it do that? | 163bae12e8c1649f03d00c75f1f14392d3a165a705e130e2c8c2ef8072900298 | [
{
"content": "I want my Python code not to error out if it runs into an error and to follow a different line of code. How do I make it do that?",
"role": "user"
},
{
"content": "One way you can accomplish that is by using a Try Except statement. This type of statement will separate your code into two blocks, a Try block and an Except block. The Try block will run the code, and if it raises an exception, it will execute the Except block. If no exception is raised, only the Try block will run. Here is an example:\n\ndef divide_numbers(x, n):\n try:\n quotient = x // n\n print(\"Answer:\", quotient)\n except:\n print(\"Cannot perform division.\")\n\nThis will run the Try block and divide x by n. If there are no errors, it will print the answer. However, if it encounters an error, it will instead print \"Cannot perform division.\" For example:\n\ndivide_numbers(6, 3)\n# Output:\n# Answer: 2\n\ndivide_numbers(2, 0)\n# Output:\n# Cannot perform divsion.\n\nYou can also specify the exception in the Except statement like this:\n\ndef divide_numbers(x, n):\n try:\n quotient = x // n\n print(\"Answer:\", quotient)\n except ZeroDivisionError:\n print(\"Cannot perform division by zero.\")\n\nThis way, the Except block will only run if ZeroDivisionError is raised. Does this help?",
"role": "assistant"
}
] | Coding |
Show me how to plot a scatterplot with seaborn in Python using my juicebar_sales.csv file, juice amount vs. sales, in an event graph with a color gradient based on juice? | 489ec38b91c95df5035c1d889c2a32ed40ca97fa306732d72589217906a120a2 | [
{
"content": "Show me how to plot a scatterplot with seaborn in Python using my juicebar_sales.csv file, juice amount vs. sales, in an event graph with a color gradient based on juice?",
"role": "user"
},
{
"content": "That's simple to do using the `seaborn` and `pandas` modules. In the code below, the two libraries are imported as `sns` and `pd`. It uses `pd.read_csv()` to read the CSV file \"juicebar_sales.csv\" and convert it to the DataFrame `df`. The scatterplot is created using `sns.scatterplot()`, specifying the data as `df`, the style as `event`, and the hue of the markers to be based on `juice_amount`.\n\n# Import the modules\nimport seaborn as sns\nimport pandas as pd\n\n# Load the CSV\ndf = pd.read_csv(\"juicebar_sales.csv\")\n\n# Create the scatter plot\nsns.scatterplot( x= \"juice amount\", y = \"sales\", data = df, style = \"event\", hue = \"juice_amount\")",
"role": "assistant"
}
] | Coding |
Could you help me come up with a descriptive comment for each line of this Typescript function?
export const pickKeys = <T, K extends keyof T = keyof T>(obj: T, ...keys: K[]): Pick<T, K> => {
return keys.reduce((acc, key) => {
if (hasKey(obj, key)) {
acc[key] = obj[key];
}
return acc;
}, {} as Pick<T, K>);
}; | a74836ebd3c90c383a4008391560ec957dfa0b5da9e29f37cbcb25aa03162300 | [
{
"content": "Could you help me come up with a descriptive comment for each line of this Typescript function?\nexport const pickKeys = <T, K extends keyof T = keyof T>(obj: T, ...keys: K[]): Pick<T, K> => {\n\treturn keys.reduce((acc, key) => {\n\t\tif (hasKey(obj, key)) {\n\t\t\tacc[key] = obj[key];\n\t\t}\n\t\treturn acc;\n\t}, {} as Pick<T, K>);\n};",
"role": "user"
},
{
"content": "Here is the Typescript pickKeys function with a descriptive comment above each line:\n\n// Accepts an object followed by any number of keys of the object.\nexport const pickKeys = <T, K extends keyof T = keyof T>(obj: T, ...keys: K[]): Pick<T, K> => {\n // Returns an object produced from mapping over each key.\n return keys.reduce((acc, key) => {\n // Checks if the current key is present in the object.\n if (hasKey(obj, key)) {\n // Sets that key in the accumulator to its value in the object if it is present.\n acc[key] = obj[key];\n }\n // Returns the accumulator.\n return acc;\n // Provides an object as the base case for the mapping, asserting that its type is a subset of T whose keys are only the members of K.\n }, {} as Pick<T, K>);\n};",
"role": "assistant"
}
] | Coding |
Create a python script that, when given a set of x and y data points, provides a linear regression and curve fit. then displays the curve. | 7e5c5cd4c0281d3379244965dc6614199c6da3e959135a65dc64b8fe9bfbe3c6 | [
{
"content": "Create a python script that, when given a set of x and y data points, provides a linear regression and curve fit. then displays the curve.",
"role": "user"
},
{
"content": "No problem! Here is code that provides linear regression of a set of data points and performs a curve fit to the data:\n\nimport numpy as np\nimport matplotlib.pyplot as plt\n\n# Generate sample data\nx_data = np.linspace(0, 10, 50)\ny_data = 2 * x_data + 1 + np.random.normal(0, 1, size=x_data.shape)\n\n# Perform linear regression\nregression_params = np.polyfit(x_data, y_data, 1)\n\n# Perform curve fitting\nfit_params = np.polyfit(x_data, y_data, 2)\n\n# Generate points for the fitted curve\nx_fit = np.linspace(0, 10, 100)\ny_fit = np.polyval(fit_params, x_fit)\n\n# Plot the original data, regression line, and fitted curve\nplt.scatter(x_data, y_data, label='Data')\nplt.plot(x_data, np.polyval(regression_params, x_data), 'r-', label='Linear Regression')\nplt.plot(x_fit, y_fit, 'g-', label='Curve Fit')\nplt.xlabel('x')\nplt.ylabel('y')\nplt.legend()\nplt.grid(True)\nplt.show()\n\nHere we can see example data points x and y data being created by creating two arrays of random numbers, then both the curve fitting and linear regression using the function \"np.polyfit\". Finally, the code displays the results using \"matploit.pyplot\" as \"plt\".",
"role": "assistant"
}
] | Coding |
I want you to build me a simple GUI app in Java that doesn't require any dependencies. To start, I just want to see some boilerplate that shows a window with a string displaying. | 3efd76547ebd59f65357d2040a3b63b92ada7655b9d2c4de25872503038cb13e | [
{
"content": "I want you to build me a simple GUI app in Java that doesn't require any dependencies. To start, I just want to see some boilerplate that shows a window with a string displaying.",
"role": "user"
},
{
"content": "Sure! This is a sample program that uses Swing to show a window with a text label.\n\nimport javax.swing.JFrame;\nimport javax.swing.JLabel;\n\nimport java.awt.Dimension;\n\npublic class MyApp {\n private static void init(String text) {\n JFrame appFrame = new JFrame(text);\n appFrame.setDefaultCloseOperation(JFrame.EXIT_ON_CLOSE);\n appFrame.setMinimumSize(new Dimension(600, 250)); //Set a minimum width and height for our window.\n JLabel textLabel = new JLabel(text);\n appFrame.add(textLabel);\n appFrame.pack();\n appFrame.setVisible(true);\n }\n\n public static void main(String[] args) {\n init(args.length > 0 ? args[0] : \"Hello, world!\");\n }\n}\n\nWe start by importing JFrame and JLabel from Swing, which we use to display the window and text, respectively. We take whatever command line argument is given, if any (defaulting to Hello, world!), and we pass it to init, which initializes our GUI. We create a frame, which is our window here, and pass it the text to put it in the title bar. We then make it so that when we close the window, the program exits. We set a minimum size, which is totally optional, using the Dimension class. Then, we create a label and use the pack method to help us with formatting. Finally, we set the window to be visible.",
"role": "assistant"
}
] | Coding |
Explain what this Bash script does in one sentence
ps aux --sort=-%mem | head -n $((num_processes + 1)) | 1b8ef3dce83ad90f2c1a18eb70b97ec2cbc3c25663448c05014503d1a4fd3ed5 | [
{
"content": "Explain what this Bash script does in one sentence\nps aux --sort=-%mem | head -n $((num_processes + 1))",
"role": "user"
},
{
"content": "This script lists out all currently running processes, sorted from greatest memory usage to least memory usage.",
"role": "assistant"
}
] | Coding |
Help me figure out what's wrong with the Python code below. It's supposed to count the number of characters that overlap between the beginning of one string and the end of another. overlap('CTAAGT', 'TTCA') should return 1, but instead it returns 2.
def overlap(string1, string2):
if len(string2) < len(string1):
temp = string1
string1 = string2
string2 = temp
print("string1: " + string1)
print("string2: " + string2)
for i in range(0, len(string1) + 1):
print(f'{string1[i:]} == {string2[:(len(string1) - i)]}')
if (string1[i:] == string2[:(len(string1) - i)]):
break
for j in range(0, len(string1) + 1):
print(f'{string2[j:]} == {string1[:(len(string2) - j)]}')
if (string2[j:] == string1[:(len(string2) - j)]):
break
return max(len(string1) - i, len(string2) - j) | c2abe99df0a43a558ebb63151afd3f8a96f884b33495437b5917b0d6099fd599 | [
{
"content": "Help me figure out what's wrong with the Python code below. It's supposed to count the number of characters that overlap between the beginning of one string and the end of another. overlap('CTAAGT', 'TTCA') should return 1, but instead it returns 2.\n\ndef overlap(string1, string2):\n if len(string2) < len(string1):\n temp = string1\n string1 = string2\n string2 = temp\n\n print(\"string1: \" + string1)\n print(\"string2: \" + string2)\n \n for i in range(0, len(string1) + 1):\n print(f'{string1[i:]} == {string2[:(len(string1) - i)]}')\n if (string1[i:] == string2[:(len(string1) - i)]):\n break\n\n for j in range(0, len(string1) + 1):\n print(f'{string2[j:]} == {string1[:(len(string2) - j)]}')\n if (string2[j:] == string1[:(len(string2) - j)]):\n break\n\n return max(len(string1) - i, len(string2) - j)",
"role": "user"
},
{
"content": "The second for loop ends too early before it checks every position in the longer string to see if an overlap starts there. If you replace the len(string1) with len(string2) in the range of the for loop, it will continue iterating until it finds the real overlap value. Here's the updated code:\n\ndef overlap(string1, string2):\n if len(string2) < len(string1):\n temp = string1\n string1 = string2\n string2 = temp\n\n print(\"string1: \" + string1)\n print(\"string2: \" + string2)\n \n for i in range(0, len(string1) + 1):\n print(f'{string1[i:]} == {string2[:(len(string1) - i)]}')\n if (string1[i:] == string2[:(len(string1) - i)]):\n break\n\n for j in range(0, len(string2) + 1): # CHANGE: len(string1) to len(string2)\n print(f'{string2[j:]} == {string1[:(len(string2) - j)]}')\n if (string2[j:] == string1[:(len(string2) - j)]):\n break\n\n return max(len(string1) - i, len(string2) - j)",
"role": "assistant"
}
] | Coding |
May you write me an example snippet of code that uses the animation library framer motion? | 65b835d2335e23cf908b10817be1eb5c1bd5dcd3b80bd3e7a46c72d2cd479e8d | [
{
"content": "May you write me an example snippet of code that uses the animation library framer motion?",
"role": "user"
},
{
"content": "Certainly, below is an example of a simple motion.div from the library framer motion being used to animate a paragraph in a React application. Motion div is one of many available tools offered by the library framer motion to allow for the seamless animation of elements on the webpage.\n\nimport {motion} from 'framer-motion';\n\nfunction App() {\n return (\n <motion.div initial={{ opacity: 0}} animate={{ opacity: 1}} transition={{ duration: 0.6 }}>\n <p>\n Lorem ipsum dolor sit amet, consectetur adipiscing elit, sed do eiusmod tempor incididunt ut labore et dolore magna aliqua. Ut enim ad minim veniam, quis nostrud exercitation ullamco laboris nisi \n ut aliquip ex ea commodo consequat. Duis aute irure dolor in reprehenderit in voluptate velit esse cillum dolore eu fugiat nulla pariatur. Excepteur sint occaecat cupidatat non proident, sunt in culpa \n qui officia deserunt mollit anim id est laborum..\n </p>\n </motion.div>\n );\n}\n\nexport default App;",
"role": "assistant"
}
] | Coding |